In previous articles we have looked at the various layout types that Android provides us. In this series of articles we’re going to have a look at a slightly more complex widget: ListView. ListView is somewhat different from the basic layouts because whereas the simple layout types offer basic layout capabilities, ListView goes further because it provides us with some pretty neat data binding facilities.
Let’s jump straight in with some code. First let’s create a new 2.3.3 project named “ListView” with a package name of “com.stylingandroid.listview” and with a main Activity named “ListViewActivity”. The first thing that we’re going to do is modify our ListViewActivity class and change it to extend ListActivity rather than Activity:
[java] package com.stylingandroid.listview;import android.app.ListActivity;
import android.os.Bundle;
public class ListViewActivity extends ListActivity
{
@Override
public void onCreate( Bundle savedInstanceState )
{
super.onCreate( savedInstanceState );
setContentView( R.layout.main );
}
}
[/java]
ListActivity is an extension of Activity which is specifically designed to host a ListView and gives us some pretty useful functionality right out of the box. It is not compulsory to host a ListView inside a ListActivity, but we’re going to use it here because it allows us to explore what it offers us.
The next thing that we need to do is to is to change the default layout in res/layout/main.xml:
[xml][/xml]
There are two key things going on here. The first is that we are declaring the ListView widget, but take note of the id that we’ve assigned it. Rather than creating an id as we usually would, we’re using an id defined by Android: android.R.id.list. The reason for this is that ListActivity will find this and automatically attach it to the Activity saving us some work. The second thing is that we’re defining a TextView (again with a specific Android id: android.R.id.empty) which ListAdapter will use when the ListView does not contain any data.
This is the first example of what ListAdapter gives us. If we run this, the TextView is displayed, and the ListView is hidden because we have not populated the ListView with any data, and the ListActivity automatically detects and manages this behaviour for us:
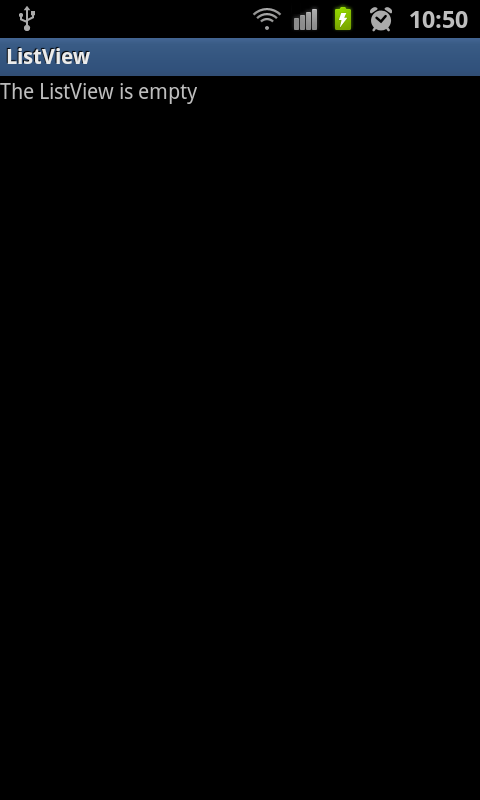
OK, then next thing to do is to bind some data to our ListView. Android defines a whole series of Adapter classes (which extends android.widget.BaseAdapter) that allow us to bind our data to controls. We need to use one of these Adapters. Let’s start by using SimpleAdapter which, as it’s name suggests, is a simple way of binding a list of objects to a control. Left’s modify the onCreate() method of ListViewActivity:
[java] @Overridepublic void onCreate( Bundle savedInstanceState )
{
super.onCreate( savedInstanceState );
setContentView( R.layout.main );
List
String[] from = { “name” };
int[] to = { android.R.id.text1 };
SimpleAdapter adapter = new SimpleAdapter( this, data,
android.R.layout.simple_list_item_1, from, to );
setListAdapter( adapter );
}
[/java]
SimpleAdapter takes five arguments:
- A Context object, so we pass in the current Context using this.
- The data which needs to be a List of Map objects. Each item in the List represents an item or row that will appear in the ListView. Each of these items is represented by a Map which links the actual data (the value of the Map entry) to a logical name (the key of the Map enty). In this case we’re using a single key called “name” which we’ll refer to in another argument.
- A resource ID of the layout to use for each item in the ListView. In this case we’re using a stock Android layout.
- A String array representing the keys to look up for each item in the data. In this case we’re creating a String array containing a single string which matches the key that we used in our data.
- An array of int values which represent the IDs of the controls to bind the data to. In this case we’re using android.R.id.text1, which is the ID of the single TextView inside the layout android.R.layout.simple_list_item_1 that we specified in argument 3.
So, argument 2 is our data (we’ve just put together some sample data here, for clarity), argument 3 is that layout to use for each item, and arguments 4 & 5 define the mapping between the data (argument 4), and the control to bind it to (argument 5).
If we run this, we can see out ListView is now populated:
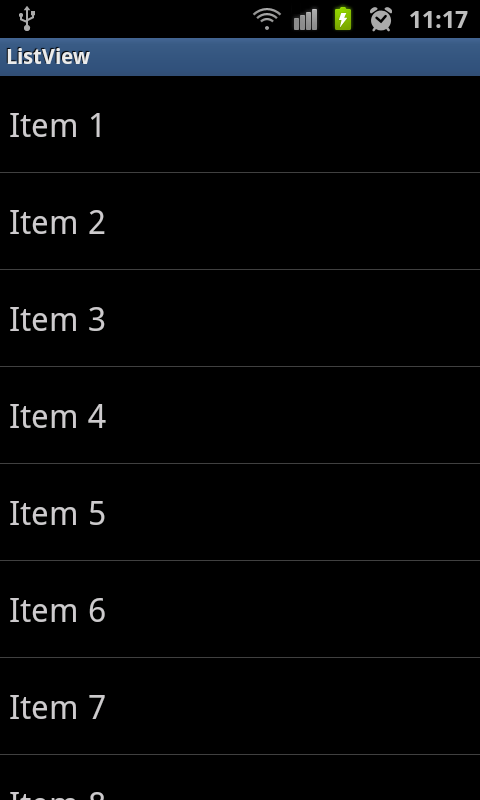
So that was a fairly simple introduction to ListView. The combination of ListView, ListActivity, and SimpleAdapter do a lot of heavy lifting for us and enable us to get a ListView working with very little code. In the next article in this series, we’ll look at handling item clicks, and life without ListActivity.
The source code fro this article can be found here.
© 2011, Mark Allison. All rights reserved.
Copyright © 2011 Styling Android. All Rights Reserved.
Information about how to reuse or republish this work may be available at http://blog.stylingandroid.com/license-information.
This can be good for the list of searched data, eg from the sql database.
Nice guide! I I didn’t realise about the handling of the case with no data.
sir., what i have two strings. here is the code.
adapter = new SimpleAdapter(this, songsListData,
R.layout.playlist_item, new String[] { “songTitle”, “songPath”}, new int[] {
R.id.songTitle, R.id.songPath});
and on the onItemClicked of the listview,, i want to get the value of the songPath..
can you teach me how to do this?